This page describes how your Chat app can open dialogs to respond to users.
Dialogs are windowed, card-based interfaces that open from a Chat space or message. The dialog and its contents are only visible to the user that opened it.
Chat apps can use dialogs to request and collect information from Chat users, including multi-step forms. For more details on building form inputs, see Collect and process information from users.
Prerequisites
Node.js
A Google Chat app that's enabled for interactive features. To create an interactive Chat app using an HTTP service, complete this quickstart.
Python
A Google Chat app that's enabled for interactive features. To create an interactive Chat app using an HTTP service, complete this quickstart.
Java
A Google Chat app that's enabled for interactive features. To create an interactive Chat app using an HTTP service, complete this quickstart.
Apps Script
A Google Chat app that's enabled for interactive features. To create an interactive Chat app in Apps Script, complete this quickstart.
Open a dialog
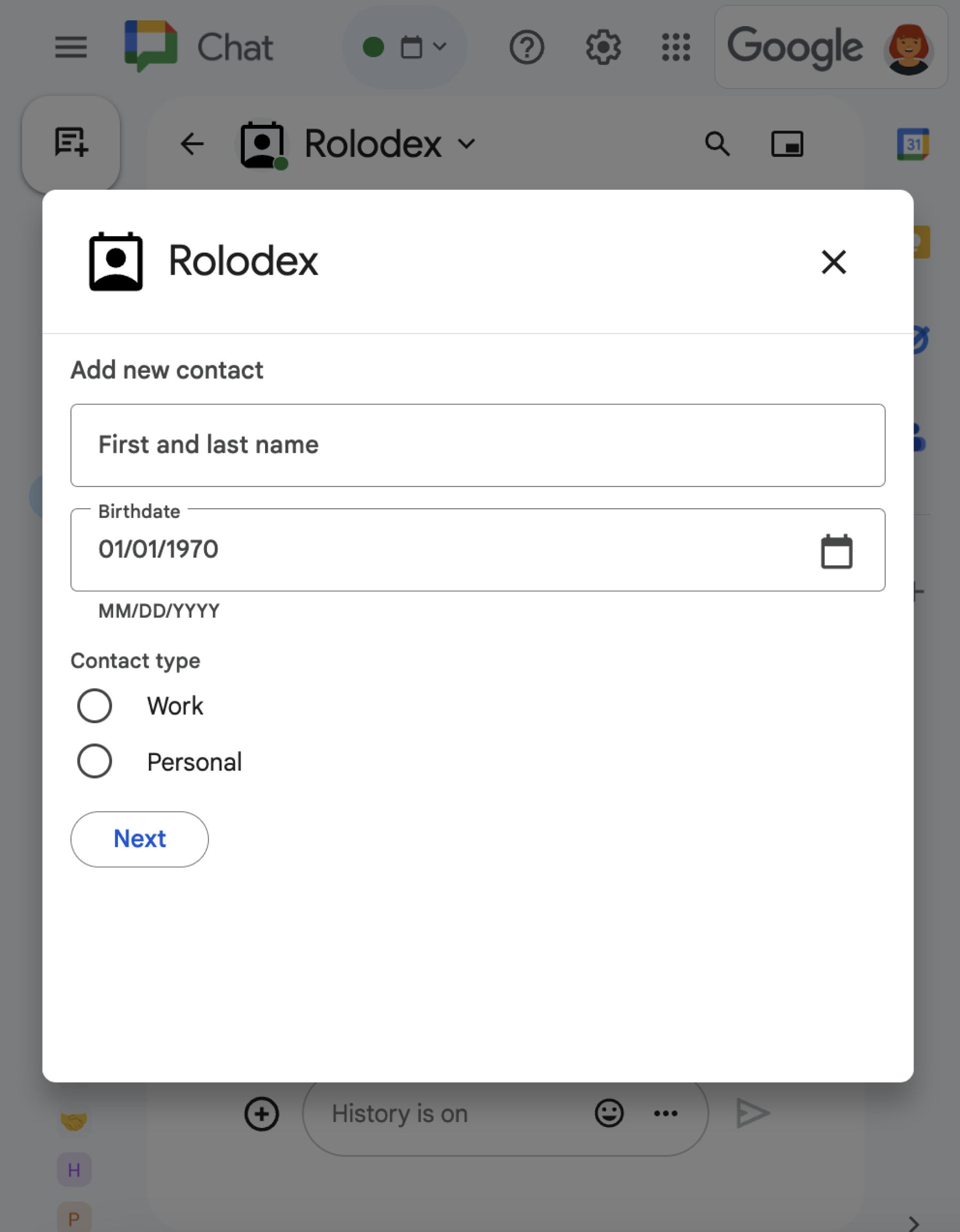
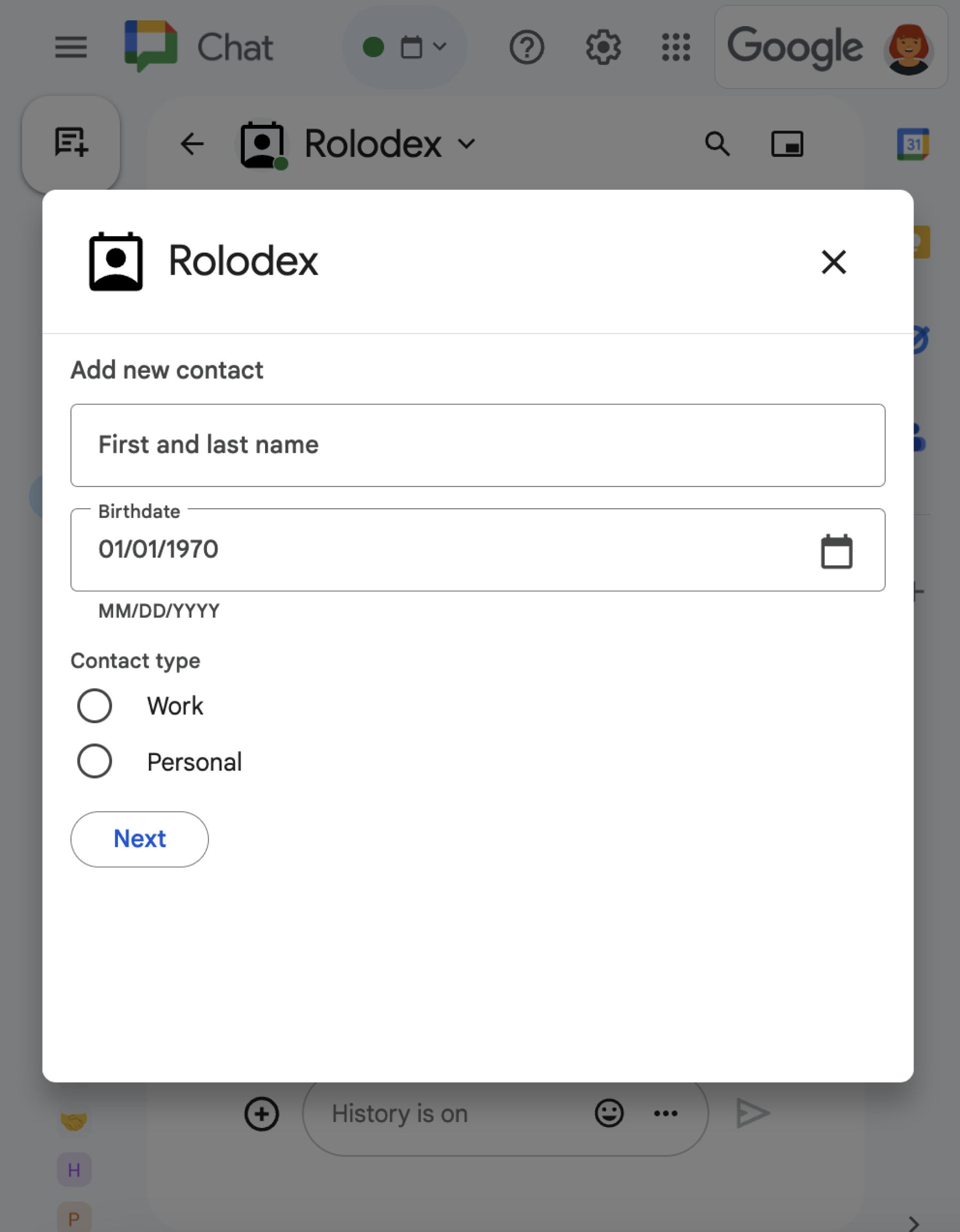
This section explains how to respond and set up a dialog by doing the following:
- Trigger the dialog request from a user interaction.
- Handle the request by returning and opening a dialog.
- After users submit information, process the submission by either closing the dialog or returning another dialog.
Trigger a dialog request
A Chat app can only open dialogs to respond to a user interaction, such as a slash command or a button click from a message in a card.
To respond to users with a dialog, a Chat app must build an interaction that triggers the dialog request, such as the following:
- Respond to a slash command. To trigger the request from a slash command, you must check the Opens a dialog checkbox when configuring the command.
- Respond to a button click in a
message,
either as part of a card or at the bottom of the message. To trigger the
request from a button in a message, you configure the
button's
onClick
action by setting itsinteraction
toOPEN_DIALOG
. - Respond to a button click in a Chat app homepage. To learn about opening dialogs from homepages, see Build a homepage for your Google Chat app.
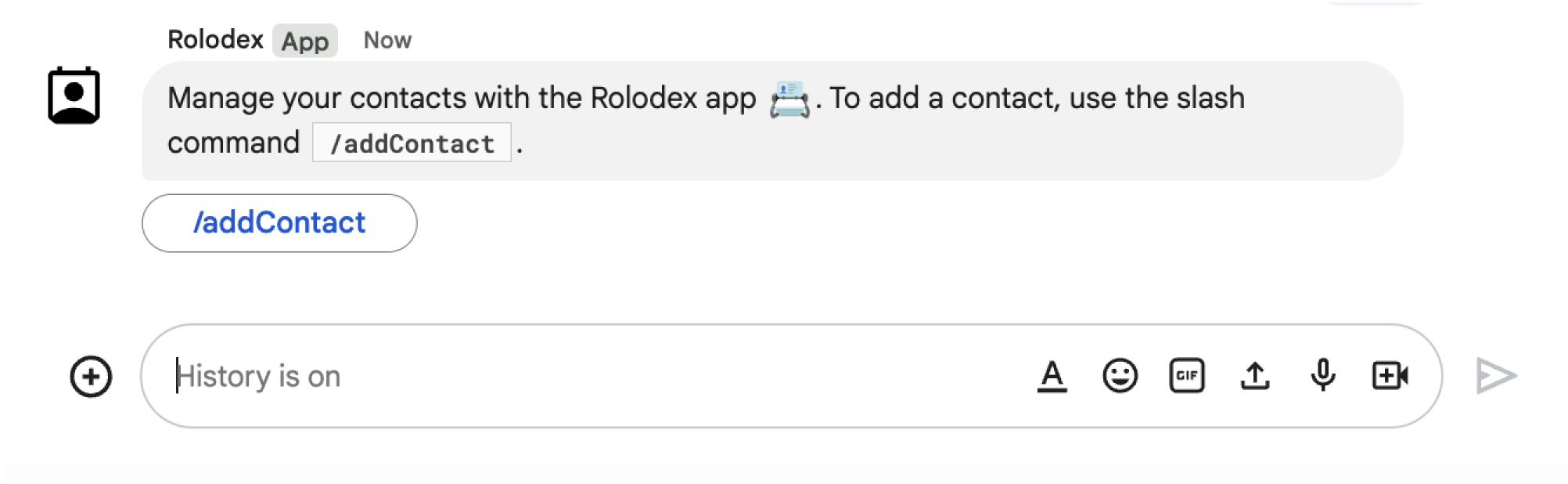
/addContact
slash command. The message also includes a button that users can click to trigger the command.
The following code sample shows how to trigger a dialog request from a button in
a card message. To open the dialog, the
button.interaction
field is set to OPEN_DIALOG
:
Node.js
Python
Java
Apps Script
This example sends a card message by returning card JSON. You can also use the Apps Script card service.
Open the initial dialog
When a user triggers a dialog request, your Chat app
receives an interaction event, represented as an
event
type in the
Chat API. If the interaction triggers a dialog request, the event's
dialogEventType
field is set to REQUEST_DIALOG
.
To open a dialog, your Chat app can respond to the
request by returning an
actionResponse
object with the type
set to DIALOG
and
Message
object. To specify the contents of the dialog, you include the following
objects:
- An
actionResponse
object, with itstype
set toDIALOG
. - A
dialogAction
object. Thebody
field contains the user interface (UI) elements to display in the card, including one or moresections
of widgets. To collect information from users, you can specify form input widgets and a button widget. To learn more about designing form inputs, see Collect and process information from users.
The following code sample shows how a Chat app returns a response that opens a dialog:
Node.js
Python
Java
Apps Script
This example sends a card message by returning card JSON. You can also use the Apps Script card service.
Handle the dialog submission
When users click a button that submits a dialog, your
Chat app receives
a CARD_CLICKED
interaction
event where the dialogEventType
is SUBMIT_DIALOG
.
Your Chat app must handle the interaction event by doing one of the following:
- Return another dialog to populate another card or form.
- Close the dialog after validating the data the user submitted, and optionally, send a confirmation message.
Optional: Return another dialog
After users submit the initial dialog, Chat apps can return one or more additional dialogs to help users review information before submitting, complete multi-step forms, or populate form content dynamically.
To process the data that users input, the Chat app
uses the
event.common.formInputs
object. To learn more about retrieving values from input widgets, see
Collect and process information from users.
To keep track of any data that users input from the initial dialog, you must add parameters to the button that opens the next dialog. For details, see Transfer data to another card.
In this example, a Chat app opens an initial dialog that leads to a second dialog for confirmation before submitting:
Node.js
Python
Java
Apps Script
This example sends a card message by returning card JSON. You can also use the Apps Script card service.
Close the dialog
When users click a button on a dialog, your Chat app executes its associated action and provides the event object with the following information:
eventType
isCARD_CLICKED
.dialogEventType
isSUBMIT_DIALOG
.
The Chat app should return an
ActionResponse
object with its type
set to DIALOG
and dialogAction
.
Optional: Display a notification
When you close the dialog, you can also display a text notification.
The Chat app can respond with a success or error
notification by returning an
ActionResponse
with actionStatus
set.
The following example checks that parameters are valid and closes the dialog with text notification depending on the outcome:
Node.js
Python
Java
Apps Script
This example sends a card message by returning card JSON. You can also use the Apps Script card service.
For details about passing parameters between dialogs, see Transfer data to another card.
Optional: Send a confirmation message
When you close the dialog, you can also send a new message, or update an existing one.
To send a new message, return an
ActionResponse
object with the type
set to NEW_MESSAGE
. The following example closes the
dialog with text notification and confirmation text message:
Node.js
Python
Java
Apps Script
This example sends a card message by returning card JSON. You can also use the Apps Script card service.
To update a message, return an actionResponse
object that contains the
updated message and sets the type
to one of the following:
UPDATE_MESSAGE
: Updates the message that triggered the dialog request.UPDATE_USER_MESSAGE_CARDS
: Updates the card from a link preview.
Troubleshoot
When a Google Chat app or card returns an error, the Chat interface surfaces a message saying "Something went wrong." or "Unable to process your request." Sometimes the Chat UI doesn't display any error message, but the Chat app or card produces an unexpected result; for example, a card message might not appear.
Although an error message might not display in the Chat UI, descriptive error messages and log data are available to help you fix errors when error logging for Chat apps is turned on. For help viewing, debugging, and fixing errors, see Troubleshoot and fix Google Chat errors.
Related topics
- View the Contact Manager sample, which is a Chat app that uses dialogs to collect contact information.
- Open dialogs from a Google Chat app homepage.
- Set up and respond to slash commands
- Process information inputted by users